php
PHP WITH OOPS CONCEPT
Object-oriented programming is a programming model organized around Object rather than the actions and data rather than logic.
Class:
A class is an entity that determines how an object will behave and what the object will contain. In other words, it is a blueprint or a set of instruction to build a specific type of object.
In PHP, declare a class using the class keyword, followed by the name of the class and a set of curly braces ({}).
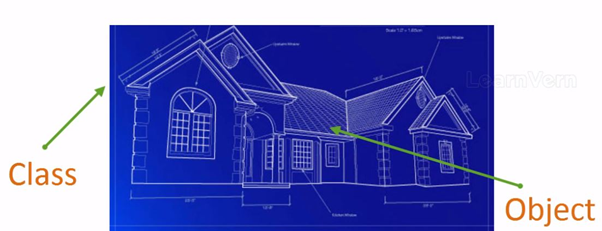
This is the blueprint of the construction work that is class, and the houses and apartments made by this blueprint are the objects.
Syntax to Create Class in PHP
- <?php
- class MyClass
- {
- // Class properties and methods go here
- }
- ?>
Important note:
In PHP, to see the contents of the class, use var_dump(). The var_dump() function is used to display the structured information (type and value) about one or more variables.
Syntax:
- var_dump($obj);
Object:
A class defines an individual instance of the data structure. We define a class once and then make many objects that belong to it. Objects are also known as an instance.
An object is something that can perform a set of related activities.
Syntax:
- <?php
- class MyClass
- {
- // Class properties and methods go here
- }
- $obj = new MyClass;
- var_dump($obj);
- ?>
Example of class and object:
- <?php
- class demo
- {
- private $a= "hello javatpoint";
- public function display()
- {
- echo $this->a;
- }
- }
- $obj = new demo();
- $obj->display();
- ?>
ABSTRACT CLASS
An abstract class is a mix between an interface and a class. It can be define functionality as well as interface.
- Classes extending an abstract class must implement all of the abstract methods defined in the abstract class.
- An abstract class is declared the same way as classes with the addition of the 'abstract' keyword.
SYNTAX:
- abstract class MyAbstract
- {
- //Methods
- }
- //And is attached to a class using the extends keyword.
- class Myclass extends MyAbstract
- {
- //class methods
- }
Example 1
- <?php
- abstract class a
- {
- abstract public function dis1();
- abstract public function dis2();
- }
- class b extends a
- {
- public function dis1()
- {
- echo "javatpoint";
- }
- public function dis2()
- {
- echo "SSSIT";
- }
- }
- $obj = new b();
- $obj->dis1();
- $obj->dis2();
- ?>
Access Specifiers in PHP
There are 3 types of Access Specifiers available in PHP, Public, Private and Protected.
Public - class members with this access modifier will be publicly accessible from anywhere, even from outside the scope of the class.
Private - class members with this keyword will be accessed within the class itself. It protects members from outside class access with the reference of the class instance.
Protected - same as private, except by allowing subclasses to access protected superclass members.
EXAMPLE 1: Public
- <?php
- class demo
- {
- public $name="Ajeet";
- functiondisp()
- {
- echo $this->name."<br/>";
- }
- }
- class child extends demo
- {
- function show()
- {
- echo $this->name;
- }
- }
- $obj= new child;
- echo $obj->name."<br/>";
- $obj->disp();
- $obj->show();
Comments
Post a Comment